In this and in a few upcoming articles, I am going to discuss Splitting Razor Components in Blazor Application. Please read our previous article, where we discussed ASP.NET Core Blazor Components in detail. As part of this article, we are going to discuss the following pointers.
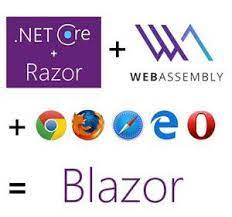
- How to Split the Razor Component in Blazor Application?
- Single file or Mixed file approach
- Partial Files Approach
- Base Class Approach
How to Split the Razor Component in Blazor Application?
In the previous Video, we discussed the Razor components in detail. As part of Razor components, we also discussed that a Razor component is a combination of two things i.e. HTML code and C# code. For example, please have a look at the following Counter.razor file i.e. the Counter Component.
This is fine when our component code is very less. But it is a good practice to separate the HTML code and C# code into their own files. It is not only good from a maintenance point of view, but it is also good for unit testing. Now let us proceed further and understand how to split a component in blazor application.
In ASP.NET Core Blazor Framework, in two ways we can split the component into their respective HTML and C# files.
- Partial files approach
- Base class approach
Before understanding the above two approaches let us first understand what is the Single file or Mixed file approach.
Single file or Mixed file approach
In this case, both the HTML markup and C# code are present in a single file. The example is the Counter.razor component which is created by default when we created a Blazor Server App a shown below.
Partial files approach
In this case, the Components HTML remains in the component file itself. Let us understand this with an example. Please modify the Counter.razor file code as shown below. Here, you can see the file only contains the HTML Markup.
As we already discussed in our previous Videos, when the component has compiled a class with the same name as the component file is generated. Here, the component name is Counter.razor. So, when we compiled this component a class file will be generated with the name as Counter.cs. And moreover, this generated class is a partial class.
As we are splitting the component code, so we need to move the C# code to a class with the same as i.e. Counter. So, create a class file with the name Counter.razor.cs within the Pages folder and then copy and paste the following code in it.
Once you created this, now if you look at the Pages folder, then you will see that this class is nested inside the Counter.razor component as shown below.
This is because, by default, the File nesting is enabled in Blazor application. If you dont want this, then you can disable this by clicking on the following button.
Once you disable to file nesting, then the Counter.razor.cs class file is no longer nesting inside the Counter.razor component as shown below.
With the above changes in place, now run the application and you should get the output as expected in the browser.
Base Class Approach
In order to understand the Base Class Approach, first the delete the Partial Counter class (Counter.razor.cs file) that we just created in the Pages folder. In this case, also, the HTML Markup will remain in the component file itself. Let us understand this with an example.
Create a separate class file
First, create a class file with the name CounterBase within the Pages folder and then copy and paste the following code in it. You can name the class anything you want, but it is a common convention to have the same name as the component but suffixed with the word Base.
Note: In this example, the component name is Counter. So, the class that contains the C# code is named as CounterBase. The class has inherited from the built-in ComponentBase class. This class is present in Microsoft.AspNetCore.Components namespace.
Modifying the Counter.razor component:
Modify the Counter.razor component file as shown below. Here, we need to include the CounterBase derived class. Once the component is compiled, the Counter component will become a derived class of the CounterBase base class.
At this point, if you build the solution, then you will get the following error.
This is because we have declared the method and variable as private in CounterBase class and we know that private members are not accessible to the derived classes. In order to resolve this issue, either we need to convert them into protected or public members. So, lets modify the CounterBase class as shown below.
Now, with the above changes in place and run the application and you should get the output as expected.
In the next Video, I am going to discuss Creating Model in Blazor Application. Here, in this Video, I try to explain how to split component in Blazor application. I hope you enjoy this Video.