In this article, I am going to discuss the Entity Framework Context Class with an example. Please read our previous article where we discussed the Architecture of Entity Framework in Detail. At the end of this article, you will understand what exactly the Context Class is and when and how to use this Context Class in Entity Framework with an example.
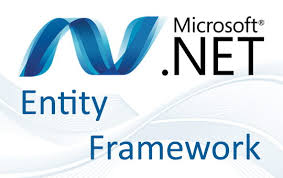
What is Context Class in Entity Framework?
The Entity Framework allows us to query, insert, update, and delete data using Common Language Runtime (CLR) objects which are also known as entities. The Entity Framework maps the entities and relationships that are defined in our model to a database.
The primary class that is responsible for interacting with data as objects is System.Data.Entity.DbContext. The context class in Entity Framework is a class that derives from DBContext in EF 6 and EF Core. It is an important class in Entity Framework, which represents a session with the underlying database.
We are going to work with the same example that we created in our Introduction to Entity Framework Video. In that console application, we retrieve the data from the SQL Server database using Entity Framework.
First, lets have a look at the solution. Please double click on the EF_Demo_DBEntities which is inside the EmployeeDataModel.Context.cs which is inside EmployeeDataModel.Context.tt file as shown below.
The following EF_Demo_DBEntities class is an example of a context class that is created by Entity Framework.
In the above example, the EF_Demo_DBEntities class is derived from DbContext class which makes it a context class. It also includes an entity set for Departments and Employees entities. The context class is used to query or save data to the database. It is also used to configure domain classes, database-related mappings, change tracking settings, caching, transactions, etc. which we will discuss in detail in our upcoming Videos.
How to query using Context Class?
In order to understand how to query using the Context class, please modify the Program class as shown below.
Run the application and see the results. We will discuss more about the Context class in a later Video.
In the next Video, I am going to discuss the Entities in Entity Framework. Here, in this Video, I try to explain the Context class in Entity Framework with one example. I hope this Video will help you with your need. I would like to have your feedback. Please post your feedback, question, or comments about this Video