The Startup class configures your applications services and defines the middleware pipeline. Generally speaking, the Program class is where you configure your applications infrastructure, such as the HTTP server, integration with IIS, and configuration sources.
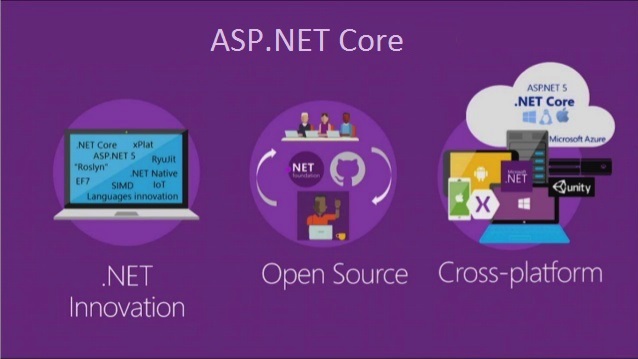
ASP.NET Core Startup Class
In this Video, I am going to discuss the ASP.NET Core Startup class in detail. Please read our previous Video where we discussed the ASP.NET Core launchSettings.json file.
Startup Class in ASP.NET Core Application:
The ASP.NET Core application must include a Startup class. It is like the Global.asax file our traditional .NET application. As the name suggests, it is executed first when the application starts. The startup class can be configured using UseStartup<T>() extension method at the time of configuring the host in the Main() method of Program class. Please have a look at the below Program class and please focus on the webBuilder.UseStartup<Startup>() method.
The name “Startup†is by the ASP.NET Core convention. However, you can give any name to the Startup class, just specify it as the generic parameter in the UseStartup<T>() method. For example, to name the Startup class as MyStartup, specify it as.UseStartup<MyStartup>().
Open Startup class in Visual Studio by clicking on the Startup.cs class file in the solution explorer. The following is a default Startup class in ASP.NET Core 3.x.
As you can see in the code, the Startup class includes two public methods: ConfigureServices and Configure. The Startup class must include the Configure method and can optionally include the ConfigureService method.
ConfigureServices() method in ASP.NET Core Startup class
The Dependency Injection pattern is used heavily in ASP.NET Core architecture. It includes the built-in IoC container to provide dependent objects using constructors.
The ConfigureServices method is a place where you can register your dependent classes with the built-in IoC container. After registering the dependent class, it can be used anywhere in the application. You just need to include it in the parameter of the constructor of a class where you want to use it. The IoC container will inject it automatically.
ASP.NET Core refers to the dependent class as a Service. So, whenever you read “Service†then understand it as a class that is going to be used in some other class.
The ConfigureServices method includes the IServiceCollection parameter to register services to the IoC container. For example, if you want to add RazorPages services or MVC services to your asp.net core application, then you need to add those services to the parameter this method accepts as shown in the below image.
Configure() method in ASP.NET Core Startup class
The Configure method is a place where we can configure the application request pipeline for our asp.net core application using the IApplicationBuilder instance that is provided by the built-in IoC container.
ASP.NET Core introduced the middleware components to define a request pipeline, which will be executed on every request. You include only those middleware components which are required by your application and thus increase the performance of your application.
The default configure method of ASP.NET Core application with the Empty template includes the following three middlewares as shown in the below image.
As we progress in this course, we will discuss more these two methods.