In this article, I am going to discuss how to implement Client Validation Using Basic Authentication in Web API. Please read our previous article before proceeding to this article as we are going to work the same example. In our last article, we discussed how to implement Token Based Authentication in ASP.NET Web API.
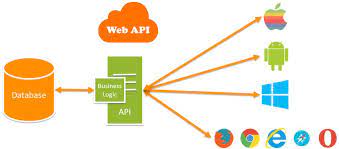
If you observed in the last Video, we have created the following MyAuthorizationServiceProvider class.
The first method i.e. ValidateClientAuthentication method is responsible for validating the Client, in the above example, we assume that we have only one client so well always return that it is validated successfully.
Lets change the requirement. Assume that we have more than one client, who is going to consume our service. In such a case, we need to validate the clients within the ValidateClientAuthentication method.
Lets see how to achieve this.
For this, we are going to use the following ClientMaster table
Please use below SQL Script to create and populate the ClientMaster table with some test data.
Create a class file with the name ClientMasterRepository.cs and then copy and paste the following code.
Here we create the ValidateClient method which is very straightforward. Its the ClientID and ClientSecret as input parameter and checks in the ClientMaster table whether the client is valid or not and it simply returns the client details.
Now we need to modify the ValidateClientAuthentication() method of MyAuthorizationServerProvider class as shown below.
Note: We need to pass the ClientId and ClientSecret using the Basic authentication in the authorization header i.e. in Base64 encoded format.
Modify the GetResource1 action method of the TestController as shown below.
Testing the API using Postman:
Lets first create the Base64 Encode value by for the ClientID and ClientSecret by using the following website
Enter the ClientID and ClientSecret separated by a colon (:) in “Encode to Base64 format†textbox, and then click on the “Encode†button as shown in the below diagram which will generate the Base64 encoded value.
Once you generate the Base64 encoded string, lets see how to use basic authentication in the header to pass the Base64 encoded value.
Here we need to use the Authorization header and the value will be the Base64 encoded string followed the “BASIC†as shown below.
Authorization: BASIC QzFBMDNCMTAtN0Q1OS00MDdBLUE5M0UtQjcxQUIxN0FEOEMyOjE3N0UzMjk1LTA2NTYtNDMxNy1CQzkxLUREMjcxQTE5QUNGRg==
Lets see step by step procedure to use the Postman to generate the Access Token
Step1:
Select the Method as POST and provide URI as shown below in the below image
Step2:
Select the Header tab and provide the Authorization value as shown below.
Authorization: BASIC QzFBMDNCMTAtN0Q1OS00MDdBLUE5M0UtQjcxQUIxN0FEOEMyOjE3N0UzMjk1LTA2NTYtNDMxNy1CQzkxLUREMjcxQTE5QUNGRg==
Step3:
Select the Body Tab. Then choose x-www-form-urlencoded option and provide the username and password value. Provide the grant_type value as password as shown in the below image,
Now click on the Send button which will generate the access token as shown below.
Once the access token is generated, we use that token to access the resources as shown below.
In the next Video, I will discuss how to generate Refresh Token in ASP.NET Web API. Here, in this Video, I try to explain how to implement Client Validation Using Basic Authentication in Web API with an example. I hope this Video will help you with your need. I would like to have your feedback. Please post your feedback, question, or comments about this Video.