In this article, I am going to discuss the Ordering Operators in LINQ with examples. Please read our previous article where we discussed the Union Method in C# with examples. At the end of this article, you will learn the following two things.
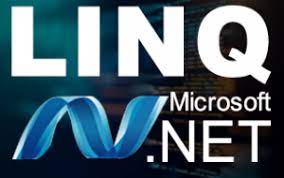
What Are Ordering Operators?
In simple terms, we can say that Ordering is nothing but a process to manage the data in a particular order. It is not changing the data or output rather this operation arranges the data in a particular order i.e. either ascending order or descending order.
In this case, the count is going to be the same but the order of the element is going to change.
We sort the data in two ways i.e. ascending or descending order. The order may be integer-based or any other data type based. For example
- Name of Cities of a particular state in alphabetical order.
- Students order by Roll Number in a class.
It is also possible to order based on multiple columns like Employee First and Last Name in ascending order while the Salary is on descending order.
What are the Methods available in Linq for Sorting the data?
There are five methods provided by LINQ to sort the data. They are as follows
- What is Linq OrderBy Method?
- Example of Linq OrderBy Method using both Method and Query Syntax.
- How to use Linq OrderBy Method with Complex Type in C#?
- How to use the OrderBy method along with the Filtering method?
What is Linq OrderBy Method?
The Linq OrderBy method in C# is used to sort the data in ascending order. The most important point that you need to keep in mind is this method is not going to change the data rather it is just changing the order of the data.
You can use the OrderBy method on any data type i.e. you can use character, string, decimal, integer, etc. Let us understand the use of the LINQ OrderBy method in C# using both query syntax and method syntax.
Example1: Working with integer data
In the below example we have a collection of integer data. And we sort the data in ascending order using the OrderBy method.
Output:
Exampe2: Working with string data.
In the below example we have a collection of string names. We then sort the data in ascending order using both method and query syntax.
Note: In query syntax, while we are sorting the data in ascending order then the use of ascending operator is optional. That means if we are not specifying anything then by default it is ascending. So the following two statements are the same.
Using LINQ OrderBy Method with Complex type:
We are going to work with the following Student class. So, create a class file with the name Student.cs and then copy and paste the following code in it.
As you can see, we created the Student class with four properties such as ID, FirstName, LastName, and Brach. We then created one method (i.e. GetAllStudents) within the same class which is going to return a list of all students.
Example3: Sorting the Data in Ascending Order
Here, we want to sort the data based on the Branch of the Student in ascending order.
Output:
Example4: Sorting with Filtering.
Now we need to fetch only the CSE branch students and then we need to sort the data based on the FirstName in ascending order.
Note: The most important point that you need to remember is, you need to use the Where method before the OrderBy method. The following example shows the above using both query and method syntax.
Output: