In this article, I am going to discuss the LINQ Except in C# with examples. Please read our previous article where we discussed the LINQ Distinct Method with examples. As part of this article, we are going to discuss the following pointers
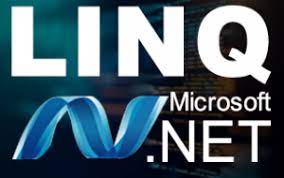
- What is LINQ Except in C#?
- Examples of C# LINQ Except Method using both Method and Query Syntax
- How to implement IEqualityComparer?
- Example using Anonymous Type
What is LINQ Except in C#?
The LINQ Except Method in C# is used to return the elements which are present in the first data source but not in the second data source. There are two overloaded versions available for the LINQ Except Method as shown below.
The one and the only difference between the above two methods is the second overloaded version takes IEqualityComparer as an argument. That means the Except Method can also be used with Comparer also.
Let us understand this with an example:
As you can see in the above image, we have two data sources i.e. DataSource 1 and Data Source 2. The DataSource 1 contains elements such as 1, 2, 3, 4, 5, 6 and the DataSource 2 contains elements such as 1, 3, 5, 8, 9, and 10. If we want to retrieve the elements such as 2, 4, and 6 from the first data source which does not exist in the second data source then we need to apply the distinct operation.
Let us see how to do this with both Query and Method syntax.
Example1:
Run the application and you will see the output as expected.
Note: In query syntax, there is no such operator call Except, so here we need to use both query and method syntax to achieve the same.
Example2:
Here we have a string array of countries and we need to return the countries from the first collection, those are not present in the second collection.
Now run the application and see the output.
In spite of having the country UK in the second collection, it still shows in the output. This is because the default comparer that is being used to filter the data is case-insensitive.
So if you want to ignore the case-sensitive then you need to use the other overloaded version of the Except() method which takes IEqualityComparer as an argument.
So, modify the program as shown where we pass StringComparer as an argument and this StringComparer class already implements the IEqualityComparer interface.
Now run the application and it will display the data as expected.
LINQ Except() Method with Complex Type:
The LINQ Except() Method in C# works slightly different manner when working with complex types such as Employee, Product, Student, etc. Let us understand this with an example.
Create a class file with the name Student.cs and then copy and paste the following code in it.
This is a very simple student class with just two properties. Let say, we have the following two data sources.
As you can see in the above image, we have two data sources. The first data source i.e. AllStudents hold the information of all the students while the second data source i.e. Class6Students hold the data of only the 6th class students.
Example3:
Our requirement is only to fetch all the student names from except the 6th class students. That means we need to fetch the student names from the AllStudents data source which are not present in the second data source i.e. Class6Students.
Output:
Example4:
Now we need to select all the information of all the students from the first data source which are not present in the second data source. Let us modify the program class as shown below.
Output:
As you can see, it displays all the students data from the first data source. This is because the default comparer which is used for comparison is only checking whether two object references are equal and not the individual property values of the complex object.
In our previous Video, we already discussed there many ways to solve the above problem. Here let us see how to use an anonymous type to solve the above problem.
Using Anonymous Type:
In this approach, we need to select all the individual properties to an anonymous type. The following program does exactly the same things.
Now run the application and it should display the output as expected. Let us see how to achieve the same thing using Comparer.
Using Comparer:
In this approach, we need to create a class and then we need to implement the IEqualityComparer interface. So, create a class file with the name StudentComparer.cs and then copy and paste the following code in it.
Then create an instance of StudentComparer class and pass that instance to the Except method as shown in the below program.
Now run the application and it should display the output as expected.