In this article, I am going to discuss the LINQ Select Projection Operator in C# with examples. Please read our previous article where we discussed what are LINQ Operators and the different categories of LINQ Operators in C#. At the end of this article, you will understand the following pointers related to Linq Select Projection Operator in C#.
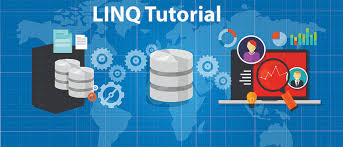
- What is Projection?
- What are Projection Operators and Methods in LINQ?
- How to use the Select Method?
- Basic Selection of Data
- How to Select Data to other classes and to anonymous type?
- Performing Calculations on the selected data using LINQ Select Operator.
- How to select data with index value?
Note: We will discuss each example using both Query and Method syntax.
What is Projection?
Projection is nothing but the mechanism which is used to select the data from a data source. You can select the data in the same form (i.e. the original data in its original state). It is also possible to create a new form of data by performing some operations on it.
What are Projection Methods or Operators available in LINQ?
There are two methods available in projection. They are as follows
- Select
- SelectMany
In this Video, we will discuss the Select Method and in the next Video, we will discuss the SelectMany Method.
Select Operator:
As we know the Select clause in SQL allows us to specify what columns we want to retrieve, whether you want to retrieve all the columns or some of the columns that you need to specify the select clause.
In the same way, the LINQ Select operator also allows us to specify what properties we want to retrieve, whether you want to retrieve all the properties or some of the properties that you need to specify in the select operator. The standard LINQ Select Operator also allows us to perform some calculations.
Example:
Let us understand the select projection operator with some examples. Here we are going to use a console application. So first create a console application with the name LINQDemo (you can give any meaningful name). Then add a new class file with the name Employee.cs. Once you add the Employee.cs class file, then copy and paste the following in it.
As you can see we have created the Employee class with the following four properties such as ID, FirstName, LastName, and Salary. We also created one static method which will return the list of employees which will act as our data source. Let us discuss some examples to understand the LINQ Select Operator.
Example1:
Select all the data from the data source using both Method and Query Syntax.
The complete code is given below.
Modify the Program class as shown below.
How to select a single property using Select Operator?
Select all the Employee IDs only using both method and query syntax.
Note: In the Query Syntax, the data type of the basicPropQuery is List<int>, this is because of the ToList() method that we applied on the query syntax. And because of this ToList() method, the query is executed at that point only.
But in the case of Method syntax, we have not applied the ToList() method and this is the reason why the data type of the basicPropMethod variable is of IEnumerable<int> type. And more importantly, at that point, the query is just generated but not executed. When we use the basicPropMethod within the foreach loop, then at that time the query is executed.
The complete example is given below.
Example3:
Our requirement is to select only the Employee First Name, Last Name, and Salary properties. We dont require to select the ID property.
The Complete code is given below.
How to Select Data to another class using LINQ Projection Operator?
It is also possible to select the data to another class using the LINQ Select operator. In our previous example, we have selected the First Name, Last Name, and Salary properties to the same Employee class. Let us create a new class with the above three properties. So, add a new class file with the name EmployeeBasicInfo.cs and then copy and paste the following code.
Now what we need to do here is, we need to project the First Name, Last Name, and Salary properties to the above newly created EmployeeBasicInfo class.
The complete example is given below.
How to Select Data to Anonymous Type using LINQ Select Operator?
Instead of projecting the data to a particular type like Employee or EmployeeBasicInfo, we can also project the data to an anonymous type in LINQ.
The complete code is given below.
How to perform calculations on data selected using the LINQ Select Operator?
Let me first explain what we want to achieve. We want to perform the following calculations
- AnnualSalary = Salary*12
- FullName = FirstName + ††+ LastName
Then we need to select the ID, AnnualSalary, and FullName to an anonymous type.
The Complete example is given below.
How to select data with index value?
It is also possible to select values using an integral index. The index is 0 based.