In this article, I am going to discuss the Angular ngFor Directive with an example. Please read our previous article where we discussed the basics of Angular Directives. The Angular Directives are one of the most important features of Angular applications. They are used to extend the power of HTML attributes. At the end of this article, you will understand what exactly angular ngFor directive is and when and how to use this directive in Angular Application.
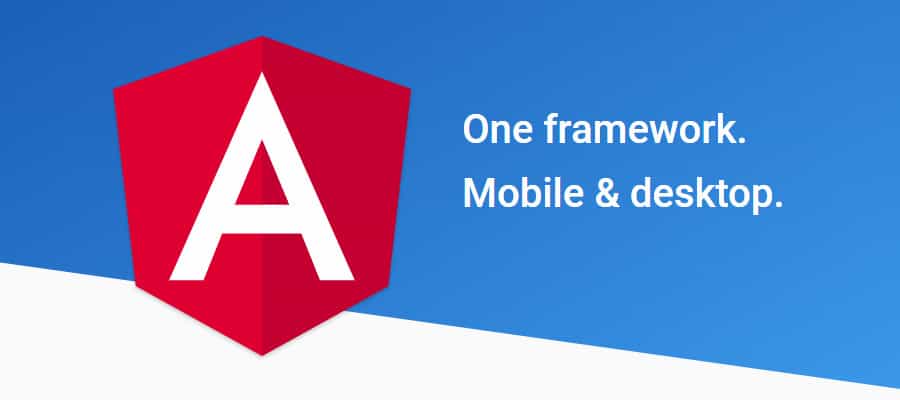
What is Angular ngFor Directive?
The built-in ngFor directive belongs to the Structural directive category. As it belongs to the structural directive category, it is used to change the structure of the DOM.
The ngFor directive is very much similar to the “for loop†used in most of the programming languages. So, the NgFor directive is used to iterate over a collection of data. The syntax to use ngFor directive is: *ngFor=â€let <value> of <collection>â€
Here, <value> is a variable name and collection is a property on your component which a collection of data. Usually an array but it can be anything that can be iterated over in a for loop.
Understanding Angular ngFor Directive:
Let us understand the ngFor structural directive in angular application with an example. We are going to use the following array of Student objects in this demo.
Then we want to display the above students in a table on a web page as shown below.
Lets discuss the Step By Step Procedure to achieve the above output using Angular ngFor Directive:
Step1: Modify the app.component.ts file
Open app.component.ts file and then copy and paste the following code in it. You can find this file within the app folder of your angular project.
As you can see in the above code, we have created one array (i.e. students) which a collection of student data. Again we have specified the templateUrl and StyleUrls, so, let proceed and modifies these two files.
Step2: Modify app.component.css file
Open app.component.css file and then copy and paste the following code in it. You can find this file within your app folder. The following styles are going to be used in our HTML page to style table data.
Step3: Modify app.component.html file
Open app.component.html file and then copy and paste the following code in it. You can also find this file within your app folder.
Understand the above Code:
- Here, the ngFor directive is used to iterate over a collection. In this example, the collection is an array of students.
- As the ngFor directive is a structural directive, so it is prefixed with * (star). So, the point that you need to remember is, all the structural directive are prefixed with a *.
- *ngFor="let student of students" – In this statement, the "student" is called template input variable, which can be accessed by the <tr> element and any of its child elements.
- The ngIf structural directive displays the row “No Students to display†when the students property does not exist or when there are no students in the array. We will discuss this directive in detail in our upcoming Videos.
Step4: Modify app.module.ts file
Open app.module.ts file which is present inside the app folder and then copy and paste the following code.
Thats it. We have done with our implementation. Now run the application and you will notice that the students are displayed in the table as expected.
ngFor – Local Variables:
The ngFor structural directive has the following local variable which you can use to customize the data.
- Index: This variable is used to provide the index position of the current element while iteration.
- First: It returns boolean true if the current element is the first element in the iteration else it will return false.
- Last: It returns boolean true if the current element is the last element in the iteration else it will return false.
- Even: It returns boolean true if the current element is even element based on the index position in the iteration else it will return false.
- Odd: It returns boolean true if the current element is an odd element based on the index position in the iteration else it will return false.
Let us understand the above ngFor local variables one by one with example.
How to get the index of an item in a collection in an angular application?
We can get the index of an item in a collection using the index property of the ngFor directive. Let us understand this with an example. What we want to do here is, along with the student data, we also want to display the index position as shown in the below image.
In order to achieve this, we want to use the ngFor local variable index. So, modify the app.component.html as shown below.
Notice that in the above code, we are using the index property of the Angular ngFor directive to store the index position of an element in a template input variable “iâ€. The variable “i†is then used in the <td> element where we want to display the index value. We used the let keyword to create the template input variable “iâ€. The let keyword in angular is very much similar to the var keyword.
The index of an element is extremely useful when you are creating the HTML elements dynamically. We will discuss creating the HTML elements dynamically in our upcoming Videos.
How to identify the first and the last elements in a collection in an angular application?
To identify the First and Last elements in a collection, you need to use the first and last properties of the ngFor directive respectively. Let us understand this with an example. We want to display the student data along with whether that student is the first or the last student as shown in the below image.
In order to achieve this, modify the app.component.html file as shown below.
How to identify the even and odd elements in a collection in an angular application?
In order to identify the Event and Odd elements in a collection in angular, you need to use the even and odd local variable of the ngFor directive respectively. Let us understand this with an example. We want to display the student data along with whether that student is odd or even as shown in the below image.
In order to achieve this, modify the app.component.html file as shown below.