In this article, I am going to discuss the Folder Structure of the Angular Application in detail. That means when we create an angular project what are different folders and files are created. What are the need and use of each file and folder in an angular application? Here, we are also going to discuss the booting process of an angular application. That means when we run the angular application, what file is executed first, then what files are executed. Which file is the entry point of the angular application? Where the file reference is stored? That means in this article, we are going to discuss the overall workflow of the angular application.
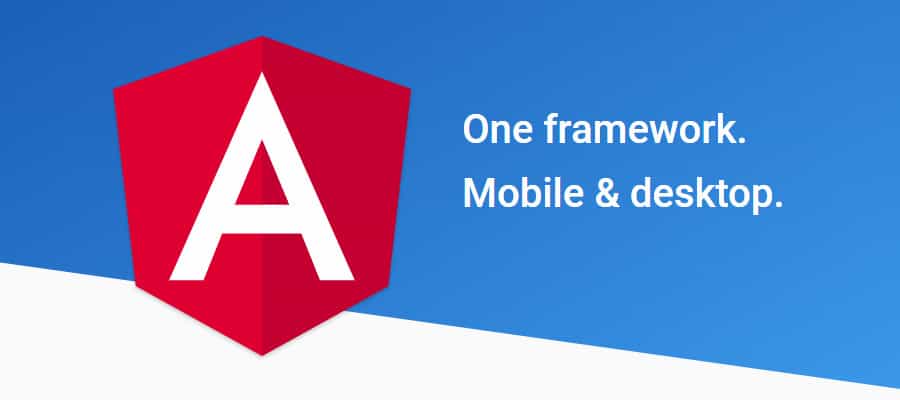
This is a continuation Video to our previous Video. So, please read our previous Video before proceeding to this Video where we discussed the step-by-step process to. Let us first open the project that we created in our previous Video using Angular CLI in the Visual Studio Code.
Opening Project using Visual Studio Code:
First launch Visual Studio Code. Then select the File => Open Folder option from the context menu as shown in the below image.
It will open the Open Folder window. Here, you need to select your project folder and then click on the select folder button as shown in the below image.
Once you click on the Select Folder button, then it will open your project in Visual Studio Code and now you can see the folder and file structure of your project as shown in the below image.
As you can see, it creates three folders (e2e, node_modules, and src) and many configuration files. Let us discuss the need and use of each folder and file in detail.
e2e Folder:
e2e stands for end-to-end testing. This is not for testing your application functionality (it is not for unit testing) rather it is used for testing the user behavior when it is deployed on the production server. That means it will monitor who visits your application, which users log in to your application, how much time they stay in your application, how many pages a user visits within your application, when the user logout from your application, etc. Please have a look at the e2e folder content.
It is not present inside the main src folder. This is because e2e tests are separate from your application. If you further notice it has its own src folder.
node_modules Folder:
This folder contains the packages (folders of the packages) which are installed into your application when you created a project in angular. If you installed any third-party packages then also their folders are going to be stored within this node_modules folder. Later in this course, we will see, when we install bootstrap, jquery like third party libraries, how the bootstrap and JQuery folders are created in this node_modules folder.
The important point that you need to remember is, you should not include this folder while you deploying your application to the production server or committing to the git repository. If you are moving your project to a new location, then also you should not include this folder and run npm which will install this folder into the new location.
src Folder:
This is the source folder of our angular application. That means this is the place where we need to put all our application source code. So, every component, service class, modules, everything we need to put in this folder only. Whenever we create an angular project, by default the angular framework creates lots of files folder within the src folder which is shown in the below image.
As you can see src folder contains many subfolders and files. Let us discuss the use and need of each subfolder and files which are present inside the src folder.
app Folder:
This is the application folder. Whenever you want to create any component, service, or module, you need to create it within this app folder. Please have a look at the following image which shows the files which are by default created within the app folder when you create an angular application. As you can see, by default it creates one component (app.component.ts) and one module (app.module.ts) and an angular application should have at least one component and one module.
Along with these two files, there are also so many other files like HTML, CSS, Sass, routing modules. We will discuss each of these files in detail in our upcoming Video.
assets Folder:
This is the folder where you can store the static assets like images, icons, etc. which needed to be copied extensively while building your application. Please have a look at the default assets folder which angular framework creates for us.
environments Folder:
This folder is basically used to set up different environments. Here, you can find two files as shown in the below image.
You can use this file to store environment (either production or development environment) specific configuration such as the database credentials or server IP addresses. These two files are as follows:
- environment.prod.ts. This file for the production environment
- environment.ts. This file for the development environment.
favicon.ico:
It is the icon file that displays on the browser.
index.html:
This HTML file contains HTML code with the head and body section. It is the starting point of your application or you can say that this is where our angular app bootstraps. If you open it you will find that there are no references to any stylesheet (CSS) nor JS files this is because all dependencies are injected during the build process.
main.ts file:
This is the entry point for our angular application. If you ever worked with programming languages like Java or C or C#, then you can compare this with the main() method of such application.
polyfills.ts
This file is basically used for browser-related configuration. In angular, you write the code using typescript language. The Polyfills.ts file is used by the compiler to compile your Typescript code to a specific JavaScript method so that it can be parsed by different browsers.
The polyfill.ts file imported the required script which is required for running an Angular application. This is because the angular framework uses the latest features of JavaScript which are not available in the current version of JavaScript supported by most of the browsers. So, basically, the polyfills.ts file fills the gap to provide the features of JavaScript that are needed by Angular and the feature supported by the latest browsers. It is mainly used for backward compatibility
.editorconfig file:
This file is basically used to set up the team environment. In real-time, many developers may work on a single project. And each developer may follow different coding standards to declare variables, classes, style, size of each character, length, etc. But in the end, we need to merge the code of each developer to produce the final product. At that time, it may produce some error or messy code as each developer having different coding standards. In order to solve this problem, the editorconfig file is used where the standard rules are defined which needs to be followed by the developers in teamwork. And moreover, the developers do not have access to this file and only the manager or the team lead who defines the rules can only have access to this file. Now, if you open the editorconfig file, you will find the following rules.
If you develop the component against the rule, then your component is not going to be compiled.
.gitignore file:
The files which you want to ignore in the git repository, you need to put within this gitignore file.
angular.json file:
From angular 6, the angular-cli.json file has been replaced by angular.json. That means if you are working with angular 5 or any lower, then you will not find this file instead you will find the angular-cli.json file.
This is one of the most important files and it contains all the configuration of your Angular Project. It contains the configurations such as what is the project name, what is the root directory as source folder (src) name which contains all the components, services, directives, pipes, what is the starting point of your angular application (index.html file), What is the starting point of typescript file (main.ts), etc. We will discuss this file in detail in our upcoming Video.
browserslist:
This file is currently used by autoprefixer to adjust CSS to support the specified browsers.
test.ts and karma.config.js
These two files are basically used for testing purposes.
- The test.ts file is the configuration file of Karma which is used for setting the test environment. Within this file, the tester will write the unit test cases for testing the project.
- The karma.config.js file is used to store the setting of karma i.e. test cases. It has a configuration for writing unit tests. Karma is the test runner and it uses jasmine as a framework. Both tester and framework are developed by the angular team for writing unit tests.
package-lock.json:
The package-lock.json is automatically generated for those operations where npm modifies either the node_modules tree or package.json. In other words, the package.lock.json is generated after an npm install. We will discuss this file in detail in our upcoming Videos.
package.json:
This file is mandatory for every npm project. It contains basic information regarding the project (name, description, license, etc), commands which can be used, dependencies – these are packages required by the application to work correctly, and dependencies – again the list of packages which are required for application however only during the development phase i.e. we need Angular CLI only during development to build a final package however for production we dont use it anymore. We will discuss this file in detail in our upcoming Videos.
README.md file:
README is the file that contains the description or description of the project which we would like to give to the end-users so that they can start using our application in a great manner. This file contains the basic documentation for your project, also contains some pre-filled CLI command information.
tsconfig.app.json:
This file is used during the compilation of your application and it contains the configuration information about how your application should be compiled.
tsconfig.json:
This file contains the configurations for typescripts. If there is a tsconfig file in a directory, that means that the directory is a root directory of a typescript project, moreover, it is also used to define different typescript compilation-related options.
tsconfig.spec.json:
This file is used for testing purposes in the node.js environment. It also helps in maintaining the details for testing.
tslint.json:
This is a tool useful for static analysis that checks our TypeScript code for readability, maintainability, and functionality errors. We will see how it works in a further lesson.