In this article, I will discuss the Route Names and Route Orders in Attribute Routing with examples. We are going to work with the same example that we worked in our previous articles. So if you have not read those articles then I strongly recommend you to read the following articles before proceeding to this article. Attribute Routing in Web API Optional URI Parameters and Default values in Attribute Routing Attribute Routing Route Prefix in WEB API Route Constraints in Attribute Routing
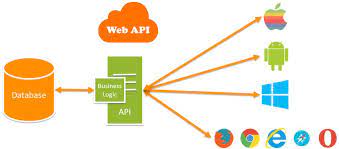
Route Names
In ASP.NET Web API, each and every route has a name. The Route names are useful for generating links so that you can include a link in an HTTP response.
To specify the route name, we need to set the Name property on the attribute. Let us see an example to understand how to set the route name, and also how to use the route name when generating a link.
Lets test this using Fiddler.
Then click on the execute button. It will give us the below result.
Route Order
When the WEB API Framework tries to match a URI with a route, it evaluates the routes in a particular order. To specify the order, set the Order property on the route attribute. Lower values are evaluated first. The default order value is zero.
Here is how the total ordering is determined:
- Compare the Order property of the route attribute.
- Look at each URI segment in the route template. For each segment, order as follows:
- Literal segments.
- Route parameters with constraints.
- Route parameters without constraints.
- Wildcard parameter segments with constraints.
- Wildcard parameter segments without constraints.
- In the case of a tie, routes are ordered by a case-insensitive ordinal string comparison (OrdinalIgnoreCase) of the route template.
Here is an example. Suppose you define the following controller:
These routes are ordered as follows.
- orders/details
- orders/{id}
- orders/{customerName}
- orders/{*date}
- orders/pending
Notice that “details†is a literal segment and appears before “{id}â€, but “pending†appears last because the Order property is 1. (This example assumes there are no customers named “details†or “pendingâ€. In general, try to avoid ambiguous routes. In this example, a better route template for GetByCustomeris “customers/{customerName}†)