In this article, I am going to discuss how to Create Custom Validation Attribute in ASP.NET MVC Application. Please read the Range Attribute in the ASP.NET MVC article before proceeding to this article as I am going to use the same example. At the moment, any value outside the range of “01/01/1970†and “01/01/2005†for DateOfBirth filed will raise a validation error.
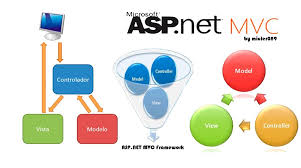
But, lets say, we want the end date to be todays date instead of the hardcode “01/01/2005†value. To achieve this we would be tempted to use DateTime.Now.ToShortDateString() as shown below.
At this point, if you compile your application, you will get the error saying – An attribute argument must be a constant expression, typeof expression or array creation expression of an attribute parameter type.
To fix this error, we can create a custom DateRangeAttribute. Here are the steps
- Right-click on the project name in solution explorer, and add “Common†folder.
- Then Right-click on the “Common†folder and add a class file with the name DateRangeAttribute.cs
- Copy and paste the following code in DateRangeAttribute.cs class file.
Finally, decorate the “DateOfBirth†property with our custom DateRangeAttribute as shown below. Notice that, we are only passing the minimum date value. The maximum date value will be todays date. Please note, DateRangeAttribute is present in MVCDemo.Common namespace.
Another example of creating a custom validation attribute in ASP.NET MVC.
Lets say our business rules have changed, and the DateOfBirth property should allow any valid date that is <= Todays Date. This means, there is no minimum value restriction and the maximum value should be less than or equal to Todays date. To achieve this, lets add another custom validation attribute. Here are the steps
- Right-click on the “Common†folder and add a class file with the name CurrentDateAttribute.cs
- Copy and paste the following code in CurrentDateAttribute.cs class file.
Decorate the “DateOfBirth“ property with our custom CurrentDateAttribute as shown below.
Please note that the validation error message can be customized using the named parameter “ErrorMessage†as shown below.