In this article, I am going to discuss customizing the auto-generated edit view in ASP.NET MVC application. This is a continuation part of our previous article, so please read our previous article before proceeding to this article. In our previous article, we discussed customizing the auto-generated Index and Create views.
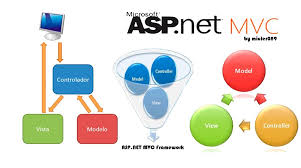
Customizing Auto-Generated Edit View
Run the application and navigate to the Index view. Click on the Edit button which will take you to edit view to edit an employee which should display as shown below.
In the edit view if you want “Select Department†as the first item in the “Department†dropdown list then replace the following line.
@Html.DropDownList(“DepartmentIdâ€,null , htmlAttributes: new { @class = “form-control†})
with
@Html.DropDownList(“DepartmentIdâ€,null , “Select Departmentâ€, htmlAttributes: new { @class = “form-control†})
Notice that a textbox is used for gender. It is ideal to have a dropdown list for gender rather than a textbox. To achieve this, make the following changes to the “Edit.cshtml†view. Replace the following code
@Html.EditorFor(model => model.Gender, new { htmlAttributes = new { @class = “form-control†} })
with
@Html.DropDownList(“Genderâ€, new List
{
new SelectListItem { Text = “Maleâ€, Value=â€Male†},
new SelectListItem { Text = “Femaleâ€, Value=â€Female†}
}, “Select Genderâ€, new { @class = “form-control†})
Creating a Field as Non Editable:
Lets make employee name as non-editable. To achieve this, change the following code in Edit.cshtml view
@Html.EditorFor(model =>model.Name)
TO
@Html.DisplayFor(model =>model.Name)
@Html.HiddenFor(model =>model.Name)
After all the modifications below is our code for Edit.cshtml view
At this point, we will still be able to change the “Name†property of the employee using tools like Fiddler. There are several ways to prevent “Name†property from being updated.
- Use UpdateModel() function and pass include and exclude list as a parameter
- Use Bind attribute
- Use Interfaces
Prevent Updating using Bind Attribute:
Now lets discuss using the BIND attribute to prevent updating the “Name†property using tools like Fiddler. Along the way, I will demonstrate adding model validation errors dynamically. Change the implementation of the “Edit†action method of Employee Controller that responds to [HttpPost] request as shown below
Here, we have not included the “Name†property from model binding using the “Bind†attribute. Even without the BIND attribute users will not be able to change the “Name†of the employee as we are copying only the required properties (Excluding name property) from the “employee†object to “employeeFromDB†which in turn is persisted to the database. Since I want to demonstrate adding model validation errors dynamically let the attribute be there.
At this point if we run the application and click on the “Save†button on the “Edit†view we get a validation error stating – The Name field is required. This is because the “Name†property is decorated with the [Required] attribute in “PEmployee.cs†file. To prevent the validation error, remove the [Required] attribute.
Now the PEmployee.cs file looks as follows
Now run the application and see Edit view is working as expected.
Adding Model Error Dynamically:
The problem with this change is that the “Name†field on the “Create†view is no longer mandatory. This means we will now be able to create a new employee without the Name value. To fix this error on the “Create†view lets add model validation errors dynamically. Change the implementation of the “Create†action method that responds to [HttpPost] request as shown below.
Thats it. Run the application and see Create View is also working as expected.