In this article, I am going to discuss the use of ViewData in ASP.NET MVC application with examples. Please read our previous article before proceeding to this article where we discussed Models in the ASP.NET MVC application. As part of this article, we are going to discuss the following pointers which are related to MVC ViewData.
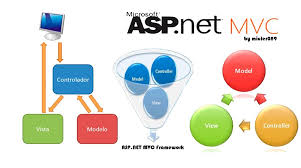
- What is ViewData in ASP.NET MVC?
- How to Pass and Retrieve data From ViewData in ASP.NET MVC?
- Example of ViewData in ASP.NET MVC.
In the ASP.NET MVC application, we can pass the model data from a controller to a view in many ways such as by using ViewBag, ViewData, TempData, Session, and Application as well as you can also use strongly typed views. You can also use the Session and Application State variable as we use in our traditional Web Forms to manage the data during a user session or throughout the application.
Now the most important question that comes to your mind is when to use ViewData, ViewBag, TempData, Session, and Application as each one having its own advantages and disadvantages. As we progress through this course you will come to know when to use one over another. Here in this Video, I will show you how to use ViewData to pass the data from a controller action method to a view.
What is ViewData in ASP.NET MVC?
The ViewData in ASP.NET MVC Framework is a mechanism to pass the data from a controller action method to a view. If you go to the definition of ViewData by right-clicking on it and select go to definition, then you will see that ViewData is defined as a property in the ConstrollerBase class and its type is ViewDataDictionary as shown in the below image.
As you can see in the above image, the return type of ViewData is ViewDataDictionary. Lets have a look at the definition of the ViewDataDictionary class.
As you can see, the ViewDataDictionary class implements the IDictionary interface. So we can say that the ViewData in ASP.NET MVC Framework is a dictionary object. As it is a dictionary object, so it is going to store the data in the form of key-value pairs where each key must be a string and the value that we are passing to the dictionary is going to be stored in the form of an object type.
How to Pass and Retrieve data From ViewData in ASP.NET MVC?
The most important point that you need to remember is, as it stores the data in the form of an object, so while retrieving the data from ViewData type casting is required. If you are accessing string data from the ViewData, then it is not required to typecast the ViewData to string type. But it is mandatory to typecast explicitly to the actual type if you are accessing data other than the string type.
ViewData in ASP.NET MVC with String Type:
ViewData in ASP.NET MVC with Complex Type:
Example of ViewData in ASP.NET MVC Application:
Let us see an example to understand how to use the ViewData to pass data from a controller action method to a view. Please read our previous Video as we are going to work with the same example. Let us first recap of what we did in our previous Video. First, we create the following Employee Model to hold the employee data in memory.
Then we created the following EmployeeBusinessLayer model to manage the employee data. Here we created one method which will take the employee id as input parameter and returns that employee information. As of now, we have hardcoded the employee data and in our upcoming Video, we will discuss retrieving the employee data from a database like SQL Server, MySQL, Oracle, etc.
Then we modify the Index action method of Home Controller as shown below to retrieve the employee data from EmployeeBusinesslayer and store it in the Employee model.
Passing ViewData From a Controller Action Method to a View:
Now we will see, how to use the ViewData to pass the employee object to the Index view. Along with we are also going to pass the page Header using ViewData. So, modify the Index action method of the Home Controller class as shown below.
Accessing ViewData in a View:
Now we will see how to access the ViewData within an ASP.NET MVC view. So, modify the Index Action method which is there within the Home folder in your application as shown below.
Thats it. Now run the application and you will see the employee details on the webpage as expected.
The ViewData in MVC is resolved dynamically at runtime. As a result, it does not provide compile-time error checking as well as we will not get the intelligence support. For example, if we miss-spell the key names then we wouldnt get any compile-time error rather we came to know the error at runtime.
The ViewData in ASP.NET MVC can only transfer the data from a controller action method to a view. That means it is valid only during the current request.