A Tag Helper Component is a Tag Helper that allows you to conditionally modify or add HTML elements from server-side code. This feature is available in ASP.NET Core 2.0 or later. ASP.NET Core includes two built-in Tag Helper Components: head and body . They're located in the Microsoft. AspNetCore.
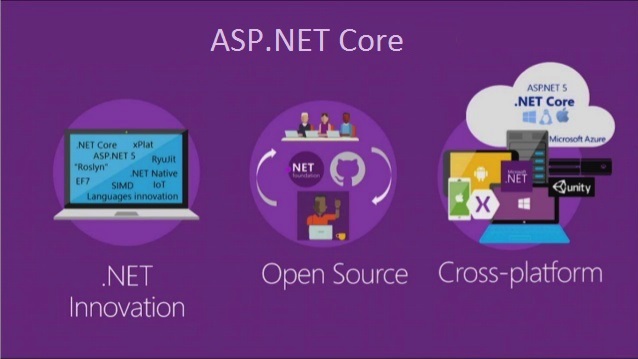
Tag Helpers in ASP.NET Core MVC
In this Video, I am going to discuss Tag Helpers in ASP.NET Core MVC Application with some examples. Tag helpers are one of the new features introduced in ASP.NET Core MVC. Please read our previous Video, where we discussed Application. As part of this Video, we are going to discuss the following pointers.
- What are Tag Helpers in ASP.NET Core?
- Types of Tag Helpers
- How to use built-in Tag Helpers in ASP.NET Core MVC?
- Understanding the Anchor Tag Helper in ASP.NET Core
- Advantage of using Tag helpers in ASP.NET Core MVC Application
What are Tag Helpers in ASP.NET Core?
Tag Helpers in ASP.NET Core are the server-side components. They are basically used to perform defined transformations on HTML Elements. As they are server-side components, so they are going to be processed on the server to create and render HTML elements in the Razor files.
If you are coming from ASP.NET MVC background, then you may be worked with the HTML helpers. The Tag Helpers are similar to the HTML helpers.
Types of Tag Helpers in ASP.NET Core:
There are two types of Tag helpers in ASP.NET Core. They are as follows:
- Built-In Tag Helpers: They come in-built in the ASP.NET Core Framework and can perform common tasks like generating links, creating forms, loading assets, showing validation messages, etc.
- Custom Tag Helper: That can be created by us to perform our desired transformation on an HTML element.
Note: In this Video, I am going to discuss the Built-in Tag Helpers and in our upcoming Videos, I will discuss the Custom Tag Helpers.
Please read the following Videos before proceeding to this Video as we are going to use layout, ViewImport, and bootstrap in this demo.
How to create and use Layout in ASP.NET Core?
Understanding the need and use of _ViewImport.cshtml file.
How to install bootstrap in ASP.NET Core?
Using Bootstrap in ASP.NET Core.
How to use built-in Tag Helpers in ASP.NET Core MVC?
In order to make the built-in tag helpers available for all the views of our application, import the tag helpers using the _ViewImports.cshtml file. You need to import them using the @addTagHelper directive as shown below.
@addTagHelper *, Microsoft.AspNetCore.Mvc.TagHelpers
The @addTagHelper makes the built-in tag helpers to available in the application which are defined in an assembly called Microsoft.AspNetCore.Mvc.TagHelpers. Here the wildcard “*†specifies that all the Tag Helpers are made available.
Generating Links using Tag Helpers in ASP.NET Core MVC Application:
Let us understand this with an example. First create a class file with the name Student.cs within the Models folder. Once you create the Student.cs class file, then copy and paste the following code in it.
Modifying Home Controller:
Modify the Home Controller as shown below. Here we have created two action methods. To make the things simple and to keep focus on Tag helpers, here we have hardcode the required student data within the action method.
Creating the Details view:
Details.cshtml:
Index View:
Now in the index view, we have to provide the View button as a link and on the click, on the View button, we need to display the Student Details. For example, we need to generate the following hyperlink. The number 101 is the ID of the student whose details we want to view.
/home/details/101
We have many different options to generate a link in ASP.NET Core MVC Application. Let us discuss all the possible options to generate a link and then we will discuss why we should use Tag Helper over others.
Option1: Manually generating the links:
Option2: Using HTML helpers
Option3: Using Tag Helpers:
In order to use Tag Helpers first import the @addTagHelper directive in the _ViewImport.cshtml file. Along with the @addTagHelper directive, we also add the model namespace using the @using directive. So, modify the _ViewImport.cshtml file as shown below which you can find within the Views folder.
Then modify the Index view as shown below.
Now run the application and it should work as expected.
Understanding the Anchor Tag Helper in ASP.NET Core:
The Anchor Tag Helper in ASP.NET Core creates the standard HTML anchor (<a … ></a>) tag by adding new attributes such as:
- asp-controller: It is used to specify the controller to target based on the routing system. If you omitted this, then the controller of the current view is used by default.
- asp-action: It is used to specify the Action method to target based on the routing system. If you omitted this attribute then the action rendering the current view is used by default.
- asp-route-{value}: It is used for specifying the additional segment value for the URL. For example, asp-route-id is used to provide value for the "id" segment.
The rendered anchor elements “href†attribute value is determined by the values of these “asp-“ attributes. As the name says, asp-controller specifies the name of the controller whereas asp-action specifies the name of the action name. Similarly, the asp-route-{value} attribute is used to include route data in the generated href attribute value. {value} can be replaced with route parameters such as id, name, etc.
Let us have a look at the following image.
As you can see in the above image, manually generating the links is much easier than using HTML Helpers or Tag Helpers. Then why should we use HTML helpers or Tag Helpers over manually generating these links in ASP.NET Core?
Advantage of using Tag helpers in ASP.NET Core MVC Application:
In ASP.NET Core MVC, the Tag Helpers generates link based on the application routing templates. That means. in future, if we change routing templates, then the link generated by tag helpers will automatically reflect those changes made to the routing templates. So, the generated links just work as expected without any trouble.
On the other hand, if we have hard-coded the URL paths manually, then we need to change the code wherever we have hardcoded the path in our application when the application routing templates change.
Lets understand this with an example
The following is the Startup class of our application. Please modify the Startup class as shown below.
As you can see in the above image, we have the route template pattern as {controller=Home}/{action=Index}/{id?}.
Generating Link Manually:
In the following code, we are manually generating the link by hard-coding the URL paths as /Home/Details.
<a href=â€/Home/Details/@student.StudentIdâ€>View</a>
Generating Link using Tag Helper:
In the following code, we are generating the link using anchor tag helper.
<a asp-controller=â€Home†asp-action=â€Details†asp-route-id=â€@student.StudentIdâ€>View</a>
As you can see with Tag Helper, we have not hardcoded the URL paths. Here, we are only specifying the controller and action names and the route parameters along with their values. When the tag helpers are executed on the server they look at the route templates and generate the correct URLs automatically.
Here, in both the techniques generate the same URL path i.e. (/Home/Details/101) and it also works with the current route template i.e. ({controller=Home}/{action=Index}/{id?})
Now let us change the routing template as shown below. Notice here in the route template, we have added the string literal “dotnetâ€.
With the above changes in place, now if you generate the link manually, then it will not work whereas if you generate the link with Tag Helpers then it will work as expected.
We also have tag helpers in ASP.NET Core to generate forms. When the form is posted back to the server, the posted form values are automatically handled and the associated validation messages are displayed. Without these tag helpers, we would have to write a lot of custom code to achieve the same. If this is not clear at the moment then dont worry we will discuss this with examples in our upcoming Videos.