In ASP.NET Core, the Route Attribute support token replacement. It means we can enclose the token (i.e. controller and action) within a pair of square-braces ([ ]). The tokens (i.e. [controller] and [action]) are then replaced with the values of controller and action method name where the route is defined.
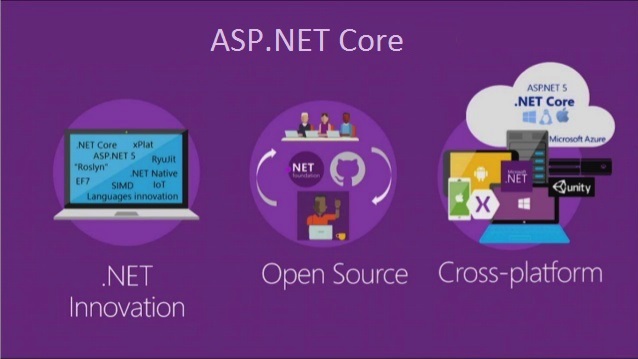
ASP.NET Core Attribute Routing using Tokens
In this Video, I am going to discuss the ASP.NET Core Attribute Routing using Tokens with examples. Please read our previous Video before proceeding to this Video as we are going to work with the same example that we worked in our previous Video. In our previous Video, we discussed Application. As part of this Video, we are going to discuss the following pointers.
- Understanding Tokens in Attribute Routing.
- Token Example in Attribute Routing.
- Advantages of using Tokens in Attribute Routing.
- Do we need to write the action token on each action method?
- Attribute Routing vs Conventional Routing in ASP.NET Core.
Tokens in Attribute Routing:
In ASP.NET Core, the Route Attribute support token replacement. It means we can enclose the token (i.e. controller and action) within a pair of square-braces ([ ]). The tokens (i.e. [controller] and [action]) are then replaced with the values of controller and action method name where the route is defined.
Token Example in Attribute Routing:
Let us understand this with an example. Please modify the Home Controller class as shown below. Here we are applying the token [controller] on the Home Controller and at the same time, we are applying the token [action] on all the action methods.
With the above controller and action tokens in place, now you can access the Index action method of Home Controller with the URL /Home/Index. Similarly, you can access the Details action method using the URL /Home/Details. Now run the application and see everything is working as expected.
Advantages of Tokens in Attribute Routing:
The main advantage is that in the future if you rename the controller name or the action method name then you do not have to change the route templates. The application is going to works with the new controller and action method names.
How to make the Index action method as the default action?
With the controller and action tokens in place, if you want to make the Index action method of Home Controller as the default action, then you need to include the Route(“â€) attribute with an empty string on the Index action method as shown below.
Do we need to write the action token on each action method?
Not Really. If you want all your action methods to apply action token, then instead of including the [action] token on each and every action method, you can apply it only once on the controller as shown below.
Attribute Routing vs Conventional Routing in ASP.NET Core:
In Attribute Routing, we need to define the routes using the Route attribute within the controller and action methods. The Attribute routing offers a bit more flexibility than conventional based routing. However, in general, the conventional based routings are useful for controllers that serve HTML pages. On the other hand, the attribute routings are useful for controllers that serve RESTful APIs.
However, there is nothing stopping you from mixing conventional based routing with attribute routing in a single application.