we can say that a ViewModel in ASP.NET Core MVC is a model that contains more than one model data required for a particular view. Combining multiple model objects into a single view model object provides us better optimization.
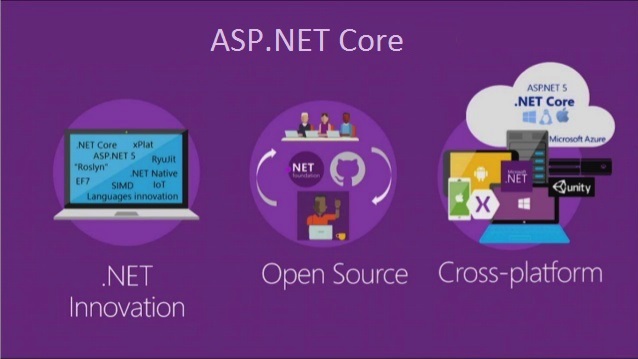
ViewModel in ASP.NET Core MVC Application
In this Video, I am going to discuss ViewModel in ASP.NET Core MVC application with an example. Please read our previous Video before proceeding to this Video where we discussed application. As part of this Video, we are going to discuss the following pointers.
- What is a View Model in ASP.NET Core?
- Why do we need the View Model?
- How to implement the View Model in ASP.NET Core Application?
What is a ViewModel in ASP.NET Core MVC?
In real-time applications, a single model object may not contain all the data required for a view. In such situations, we need to use ViewModel in the ASP.NET Core MVC application. So in simple words, we can say that a ViewModel in ASP.NET Core MVC is a model that contains more than one model data required for a particular view. Combining multiple model objects into a single view model object provides us better optimization.
Understanding the ViewModel in ASP.NET Core MVC:
The following diagram shows the visual representation of a view model in the ASP.NET Core MVC application.
Let say we want to display the student details in a view. We have two different models to represent the student data. The Student Model is used to represent the student basic details where the Address model is used to represent the address of the student. Along with the above two models, we also required some static information like page header and page title in the view. If this is our requirement then we need to create a view model let say StudentDetailsViewModel and that view model will contain both the models (Student and Address) as well as properties to store the page title and page header.
Creating the Required Models:
First, create a class file with the name Student.cs within the Models folder of your application. This is the model that is going to represent the basic information of a student such as a name, branch, section, etc. Once you create the Student.cs class file, then copy and paste the following code in it.
Next, we need to create the Address model which is going to represent the Student Address such as City, State, Country, etc. So, create a class file with the name Address.cs within the Models folder and then copy and paste the following code in it.
Creating the View Model:
Now we need to create the View Model which will store the required data that is required for a particular view. In our case its students Details view. This View Model is going to represent the Student Model + Student Address Model + Some additional data like page title and page header.
You can create the View Models anywhere in your application, but it is recommended to create all the View Models within a folder called ViewModels to keep the things organized.
So first create a folder at the root directory of your application with the name ViewModels and then create a class file with the name StudentDetailsViewModel.cs within the ViewModels folder. Once you create the StudentDetailsViewModel.cs class file, then copy and paste the following code in it.
We named the ViewModel class as StudentDetailsViewModel. Here the word Student represents the Controller name, the word Details represent the action method name within the Student Controller. As it is a view model so we prefixed the word ViewModel. Although it is not mandatory to follow this naming convention, I personally prefer it to follow this naming convention to organize view models.
Creating Student Controller:
Right-click on the Controllers folder and then add a new class file with the name StudentController.cs and then copy and paste the following code in it.
As you can see, now we are passing the view model as a parameter to the view. This is the view model that contains all the data required by the Details view. As you can notice, now we are not using any ViewData or ViewBag to pass the Page Title and Header to the view instead they are also part of the ViewModel which makes it a strongly typed view.
Creating the Details View:
First, add a folder with the name Student within the Views folder your project. Once you add the Student Folder, then you need to add a razor view file with the name Details.cshtml within the Student folder. Once you add the Details.cshtml view then copy and paste the following code in it.
Now, the Details view has access to the StudentDetailsViewModel object that we passed from the controller action method using the View() extension method. By using the @model directive, we set StudentDetailsViewModel as the Model for the Details view. Then we access Student, Address, Title, and Header using @Model property.
Now run the application, and navigate to the “/Student/Details†URL and you will see the output as expected on the webpage as shown in the below image.