Controllers are the brain of an ASP.NET Core application.They are basically C# classes whose Public methods are called as Action Methods. These Action Methods handle the HTTP requests and prepare the response to be sent to the clients
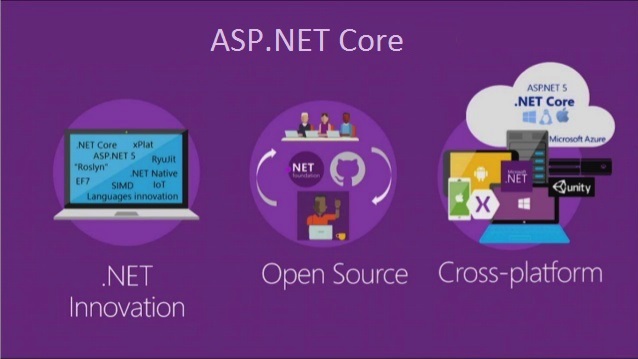
Controllers in ASP.NET Core MVC Application
In this Video, I am going to discuss the Controllers in ASP.NET Core MVC Application with an example. Please read our previous Video before proceeding to this Video where we discussed with an example. As part of this Video, we are going to discuss the following pointers.
- What is a Controller?
- Role of Controller
- How to add Controller in ASP.NET Core Application?
- What are Action Methods?
- How to call the Action method of a Controller?
- How to Pass Parameters in Action Methods?
- When should we create a new controller?
- How many controllers can we have in a single application?
What is a Controller in ASP.NET Core?
A Controller is a special class in ASP.NET Core Application with .cs (for C# language) extension. By default, when you create a new ASP.NET Core Application using Model View Controller (MVC) template, then you can see the Controllers are residing in the Controllers folder.
The Controllers in MVC application logically group similar types of actions together. This aggregation of actions or grouping similar types of action together allows us to define sets of rules such as caching, routing, and authorization which is going to be applied collectively.
In ASP.NET Core MVC Application, the controller class should and must be inherited from the Controller base class.
When the client (browser) sends a request to the server, then that request first goes through the request processing pipeline. Once the request passes the request processing pipeline, it will hit the controller. Inside the controller, there are lots of methods (called action methods) actually handle that incoming HTTP Request. The action method inside the controller executes the business logic and prepared the response which is sent back to the client who initially made the request.
Role of Controller:
- A Controller is used to group actions i.e. Action Methods.
- The Controller is responsible to handle the incoming HTTP Request.
- The Mapping of the HTTP Request is done using Routing. That is for a given HTTP Request, which action method of which controller is going to invoke is handled by the Routing Engine.
- Many important features such as Caching, Security, etc. can be applied to the controller.
How to add Controllers in ASP.NET Core Application?
If you create the ASP.NET Core Application using the MVC Project Template, then by default it will create a controller called HomeController within the Controllers folder. But if you create the ASP.NET Core Application with Empty Project template, then by default you will not find the Controllers folder in your project. As we are going to discuss everything from scratch, so we will create the ASP.NET Web Core Application with Empty Template and will add the Controllers folder and the Controllers manually.
Step1: Creating Empty ASP.NET Core Web Application
Create an ASP.NET Core Web Application with an Empty Project template. You can give any name to your application. I am giving the name for My Application as FirstCoreMVCWebApplication. The project will be created with the following folder structure and you can see there is no such folder called Controllers.
Step3: Adding Controller
Once you create the Controllers folder, next we need to add a controller (StudentController) inside this Controllers folder. To do so, right-click on the Controller folder and select the Add => Controller option from the context menu which will open the Add Controller window as shown in the below image.
As you can see in the above image, we have three templates to create an MVC controller as well as three templates to create a Web API Controller. As we are interested MVC Application, so you can use any of the following three templates:
MVC Controller – Empty: It will create an Empty Controller.
MVC Controller with read/write actions: This template will create the controller with five action methods to create, read, update, delete, and list entities.
MVC Controller with views, using Entity Framework: This template will create an MVC Controller with actions and Razor views to create, read, update, delete, and list entities using Entity framework.
Here, we are going to create the MVC Controller with the Empty template. So, select the MVC Controller – Empty option and click on the Add button as shown in the below image.
Once you click on the Add button, it will open the below window where you need to select the Controller Class – Empty option and give a meaningful name to your controller. Here, I am giving the name as StudentController and click on the Add button. Here, the point that you need to remember is the Controller names should be suffixed with the word Controller.
Once you click on the Add button then it will add StudentController within the Controllers folder as shown in the below image.
Understanding StudentController:
Now let us understand the StudentController class and the different components of this class. First of all, it is a class having a .cs extension. Open the StudnetController.cs class and you should get the following default code in it.
As you can see in the above image, the StudentController class is inherited from the Controller class, and this controller class present in Microsoft.AspNetCore.Mvc namespace and thats why it import that namespace. Now right click on the Controller and select go to the definition and you will see the following definition of Controller class.
As you can see in the above image, the Controller has many methods (Json, View, PartialView, OnActionExecuting, etc) and properties (TempData, ViewBag, ViewData, etc.) and the point that you need to remember is these methods and properties are going to used when we are working with ASP.NET Core MVC Application. Again, if you look this Controller class is inherited from the ControllerBase class.
Let us see the ControllerBase class as well. Right-click on the ControllerBase class and select go to definition and you will see the following definition. Here, you will see the RequestData, Response, Request, ModelState, Routing, Model Binder, HttpContext, and many more which we are going to use as part of our ASP.NET Core MVC Application.
Now I hope you got clarity on ASP.NET Core MVC Controllers. Let us move forward and understand the next topic which is Action Methods.
What are Action Methods?
All the public methods of a controller class are known as Action Methods. Because they are created for a specific action or operation in the application. So, a controller class can have many related action methods. For example, adding a Student is an action. Modifying the student data is another action. Deleting a student is another action. So, the point that you need to remember is all the related actions should be created inside a particular controller.
An action method can return several types. Let us modify the HomeController as shown below where we have one method which returns all the student details. Intentionally we returned a string from this method but as we progress in this course, we will discuss the real implementation of this method. But for now, just the learning purpose we have to return a string.
How to call an Action method of a Controller?
When we get an HTTP Request on a Controller, it is actually the controller action method getting that call. So, whenever we saying we are hitting a controller, it means we are hitting an action method of a controller.
The default structure is: http:domain.com/ControllerName/ActionMethodName
As we are working with development using visual studio, the domain name is going to be our localhost with some available port number. So, if we want to access the GetAllStudents action method of the HomeController then the URL is something like below.
http://localhost:<portnumber>/student/GetAllStudents
Let us prove this. At this moment if you run the application and navigate to the above URL, then you will not get the output. This is because we have created this project using the Empty template. And the Empty Project template by default will not add the required MVC Service to the built-in dependency injection container as well as it will not set the required middleware to the application processing pipeline. So, let us set these two.
Modifying the Startup class:
Open the Startup.cs class file and then copy and paste the below code in it which will add the required MVC service to the dependency injection as well as will add the MVC middleware to the request processing pipeline.
With the above change in place now run the application and navigate to the URL http://localhost:<portnumber>/student/GetAllStudents and you should get the output as expected as shown in the below image.
For a better understanding, how the above URL mapped to the GetAllStudents action method, please have a look at the following image.
How to Pass Parameters in Action Methods?
Let us understand this with an example. Now we want to search students based on the name. To do so, add the following action method inside the Student Controller.
Now run the application and navigate to the URL http://localhost:<portnumber>/student/GetStudentsByName?name=james and see the output.
In this case, the query string parameter name is mapped with the GetStudentsByName action method name parameter. We will discuss the parameter mapping, the default mapping, and many more in our upcoming Videos.
When should we create a new controller?
Whenever we need to define a new group of actions or operations into your applications, then you need to create a new controller. For example, to do operations of students you can create Student Controller. To manage the security of your application like login, logout, etc you can create a Security Controller.
How many controllers can we have in a single application?
It depends on the application. At least one controller is required to perform operations. Maximum n number of Controllers we can have into one application.